C++ Does not have string case manipulation built in to its
standard library. For this reason, it's necessary to make your own upperCase and lowerCase functions. I'll show you how to do just that.
The Function Code
For all lowercase:
void lowerCase(string& strToConvert)
{
for(unsigned int i=0;i<strToConvert.length();i++)
{
strToConvert[i] = tolower(strToConvert[i]);
}
}
For all UPPERCASE:
void upperCase(string& strToConvert)
{
for(unsigned int i=0;i<strToConvert.length();i++)
{
strToConvert[i] = toupper(strToConvert[i]);
}
}
Download the .exe and Source File
The Explanation
A string is technically an
array of characters. Although C++ can not uppercase or lowercase a string, it can uppercase or lowercase an individual character, using the toupper() and tolower() standard functions respectively. This means we need to be able to isolate the characters of a string so we can apply the toupper() or tolower() function. The way we do this is by referring to the string as an array.
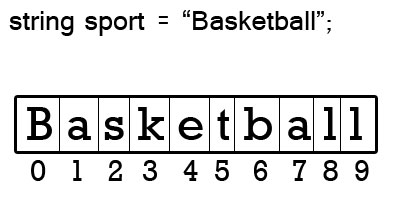
In our example of
string sport = "Basketball";
If we were to output sport[0], we would output the character 'B'.
Recall that arrays begin indexing at 0, not 1. Array indexes are contained within square brackets [ ].
If we were to output sport[3], we would output the character 'k'.
Now that we know how to isolate a character, we can apply the toupper or tolower function. As you could guess, tolower makes a character lowercase. toupper makes a character uppercase.
If we were to output tolower(sport[0]), we would get a lowercase 'b'.
If we were to output toupper(sport[5]), we would get an uppercase 'T'.
So we want to process the entire string. Since array indexes go by an increment of one, we have a perfect condition to use a for loop.
Here's our logic for this function:
- Start at the first character of the string
- Turn that character lowercase
- Move on to the next character in the string
- Repeat until all characters have been turned lowercase
This tells us
- The for loop counter should start at 0
- We should tolower the index of the string that corresponds to the counter
- The counter should be incremented by 1 after execution of the loop body
- The loop should continue until the counter reaches the length of the string
The length of the string can be found with string.length()
So the for loop turns out
for(unsigned int i=0;i<strToConvert.length();i++)
{
strToConvert[i] = tolower(strToConvert[i]);
}
Download the .exe and Source File
February 28, 2010