Greetings Canadian students. You should do your own homework!
Just kidding...eh...heh.
This Post Will Show You How To Capitalize Each Word In C++, Similar To The Way I Am Typing Right Now. This Is Also Known As Proper Case. First I Will Show You The Code, And Then Offer An Explanation.
The Code
//Joseph McCullough
//Program: capEachWord.cpp
//Description: Takes a string and capitalizes each word of the string.
//Visit www.mcculloughdesigns.com/blog for more C++ Goods!
#include <iostream>
#include <string>
using namespace std;
void lowerCase(string&);
void capEachWord(string&);
const string SENTINEL = "0";//When entered as strToConvert,
//terminates program.
int main()
{
string strToConvert; //The string that will be converted.
cout << "***********************************" << "\n"
<< "CapEachWord.exe" << "\n"
<< "Capitalizes every word in a string." << "\n"
<< "Provided By McCullough Designs" << "\n"
<< "***********************************";
//Read in strToConvert
cout << "\n\nEnter a String or enter 0 to exit: ";
getline(cin, strToConvert);
while (strToConvert != SENTINEL)
{
capEachWord(strToConvert);
cout << "\nNew String: " << strToConvert;
//Read in strToConvert
cout << "\n\nEnter a String or enter 0 to exit: ";
getline(cin, strToConvert);
}
return 0;
}
/****** function capEachWord *****
Description: converts the first letter of a word to a capital letter
PARAMETERS
strToConvert: the string being manipulated
Precondition:
strToConvert: undefined
HEADERS
#include <string>
Postcondition:
Returns the string with the first letter of every word capitalized. */
void capEachWord(string& strToConvert)
{
//Identifies if the current word has been capitalized.
//Set to false by default.
bool thisWordCapped = false;
//Turn all letters lowercase
lowerCase(strToConvert);
for (unsigned int i=0; i<strToConvert.length();i++)
{
//At a space or punctuation mark, the current word has ended.
//We are now on a new word that has not yet been capitalized,
//so thisWordCapped is set to false.
if ((ispunct(strToConvert[i])) || (isspace(strToConvert[i])))
thisWordCapped = false;
//If current word has not been capitalized AND the current character
//is a letter, uppercase the letter. The word is now capitalized, so
//thisWordCapped is set to true, and will not be set to false until
//a space or punctuation is found.
if ((thisWordCapped==false) && (isalpha(strToConvert[i])))
{
strToConvert[i]=toupper(strToConvert[i]);
thisWordCapped = true;
}
}
}
/****** function lowerCase *****
Description: makes all the characters of a string lowercase
PARAMETERS
strToConvert: the string being manipulated
Precondition:
strToConvert: undefined
HEADERS
#include <string>
Postcondition:
Returns the string all lowercase */
void lowerCase(string& strToConvert)
{
for(unsigned int i=0;i<strToConvert.length();i++)
{
strToConvert[i] = tolower(strToConvert[i]);
}
}
Download the Source Code
The Explanation
Here is a visual representation to help you understand the logic of the code.
We create a local boolean value called thisWordCapped. Like the name implies, the value is set to true when the current word of the string being processed has been capitalized. It is set to false by default, because we must account for the possibility of a letter being the first character of the string. If thisWordCapped was defaulted to true and the first character of a string was a letter, the letter would not be uppercased.
We define a word as a series of characters following a blank space or punctuation mark.
If thisWordCapped is true, no code in the loop body will execute,the counter will increment, moving us on to the next character. If thisWordCapped is false, that means the current word we are working on has not yet been capitalized. If thisWordCapped is false and the current character is a letter, we uppercase the letter and set thisWordCapped to true because we have just capitalized the word. thisWordCapped will remain true and the loop will continue to not execute anything and only increment
UNTIL we reach a space or punctuation.
Here is the logic laid out for you.
Here is our example. The string "hi. You-are nice." We have 4 words here: hi, you, are, nice. So the string should read when we're finished "Hi. You-Are Nice."
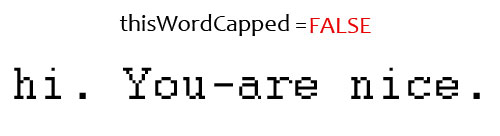
We start by lowercasing all characters using the lowerCase function.
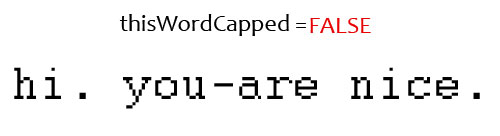
Since thisWordCapped was false and the current character is a letter, we uppercase the letter and set thisWordCapped to true.
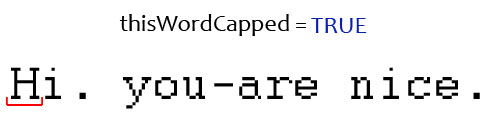
thisWordCapped is true, so we do nothing and move on to the next character.
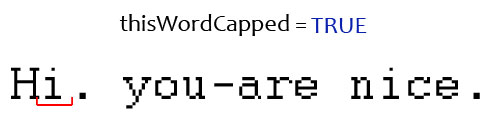
The current character is a punctuation mark, so thisWordCapped is set to false again.
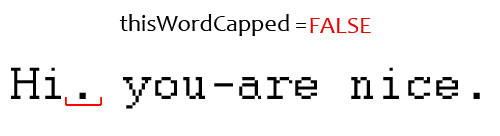
The current character is a blank space, so thisWordCapped is set to false again.
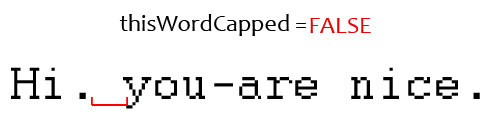
Since thisWordCapped was false and the current character is a letter, we uppercase the letter and set thisWordCapped to true.
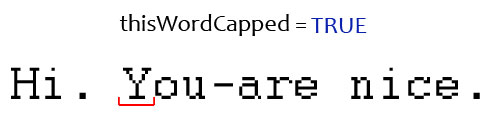
thisWordCapped is true, so we do nothing and move on to the next character.
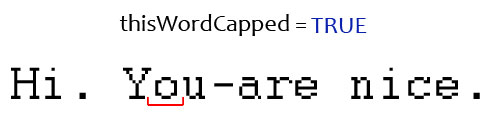
thisWordCapped is true, so we do nothing and move on to the next character.
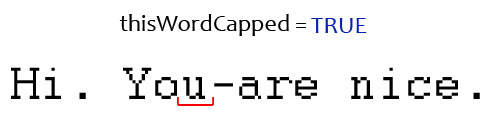
The current character is a punctuation mark, so thisWordCapped is set to false again.
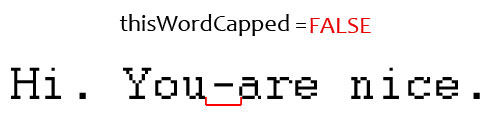
Since thisWordCapped was false and the current character is a letter, we uppercase the letter and set thisWordCapped to true.
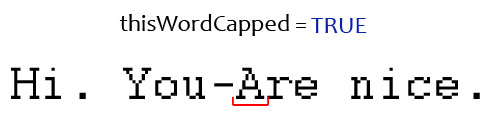
thisWordCapped is true, so we do nothing and move on to the next character.
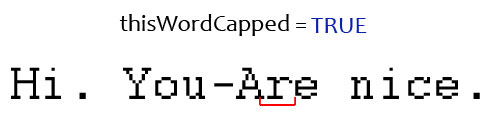
thisWordCapped is true, so we do nothing and move on to the next character.
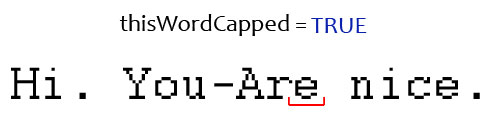
The current character is a blank space, so thisWordCapped is set to false again.
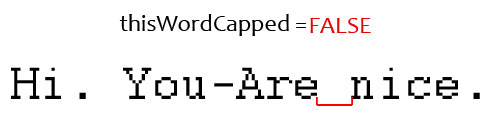
Since thisWordCapped was false and the current character is a letter, we uppercase the letter and set thisWordCapped to true.
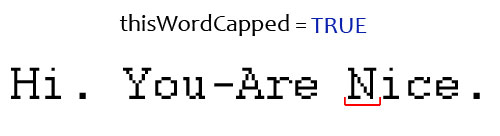
thisWordCapped is true, so we do nothing and move on to the next character.
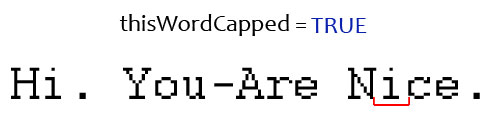
thisWordCapped is true, so we do nothing and move on to the next character.
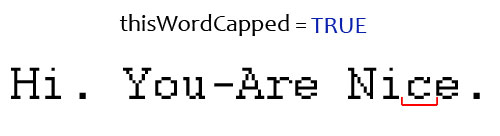
thisWordCapped is true, so we do nothing and move on to the next character.
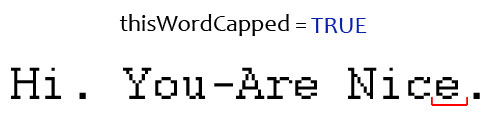
The current character is a punctuation mark, so thisWordCapped is set to false again.
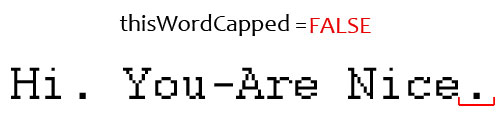
We've reached the end of the string, and this is the final result.
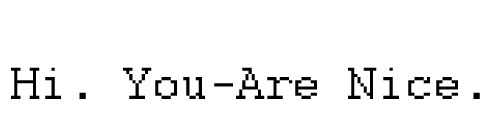
I hope the visual put things in perspective. If you are looking for how to use sentence case in C++,
visit this post.
Download the Source Code
March 04, 2010